Medium
Given the head
of a linked list, rotate the list to the right by k
places.
Example 1:
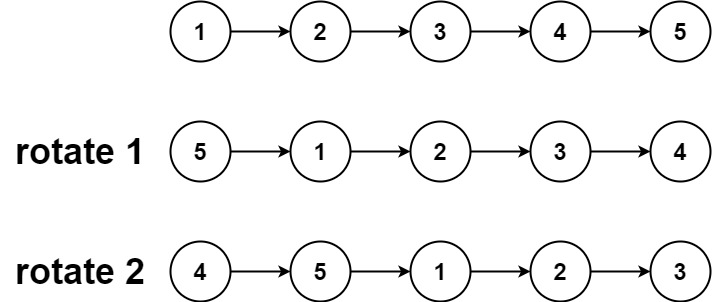
Input: head = [1,2,3,4,5], k = 2 Output: [4,5,1,2,3]
Example 2:
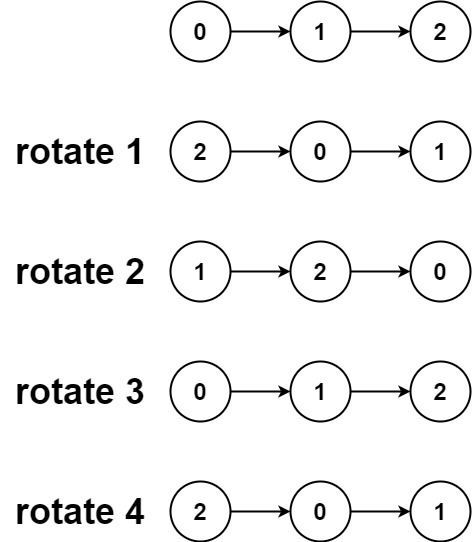
Input: head = [0,1,2], k = 4 Output: [2,0,1]
Constraints:
- The number of nodes in the list is in the range
[0, 500]
. -100 <= Node.val <= 100
0 <= k <= 2 * 109
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def get_size(self, head):
size = 1;
node = head
while node:
if node.next == None:
node.next = head
break
node = node.next
size+=1
return size
def rotateRight(self, head: Optional[ListNode], k: int) -> Optional[ListNode]:
if k == 0:
return head
if not head:
return head
elif not head.next:
return head
size = self.get_size(head)
target_pos = size - (k % size)-1
node = head
for i in range(target_pos):
node = node.next
new_head = node.next
node.next = None
return new_head